Blackjack Programming Assignment
The Python Programming class that I taught in the spring 2009 semester studied how to program graphical user interfaces. The final project of the class was to design an object oriented black jack card game with a graphical user interface. Described on the following pages is my implementation. This class was taught as a lower level CMST elective.
- Hello everyone, I would like some help in programming a blackjack python assignment. Blackjack, also know as twenty-one, is a card game that is played at casinos around the world. In casinos, players bet money on each hand (with, as you would expect in a casino, the odds stacked in favor of the dealer).
- The Card Game Assignment: Demonstration Applets Source Code Documents: Introduction. Back in the 'good ol' days' of programming, where everything was done from the command line, a card game program looked like this: Welcome to Blackjack! You have been dealt the seven of clubs. You have been dealt the six of hearts. Hit or Stand (H/S)?
Introduction
Back in the 'good ol' days' of programming, where everything was done from the command line, a card game program looked like this:
Welcome to Blackjack!
You have been dealt the seven of clubs.
You have been dealt the six of hearts.
Hit or Stand (H/S)?
Fortunately, we now have graphical user interfaces and languages such as Java that makes it easy to work with images - such as cards - in programs. Unfortunately, there is a major problem with making this type of assignment: obtaining card images to be used in the program. Scanning cards is a no-no: as the 'fine print' provided by The United States Playing Card Company puts it:
COPYRIGHT INFORMATION
BICYCLE®, BEE®, AVIATOR®, HOYLE®, CLUB SPECIAL®, the Rider Back Design, the Diamond
Back Design, the Goddess of Liberty, and other brand names, card back designs and
designs of Aces and Jokers used on this site are registered trademarks of The United
States Playing Card Company in the United States and other countries.
Therefore, in order to assign a graphically-oriented card game, one must have access to a set of cards that are not encumbered by any copyright restrictions.
If we take an object-oriented approach to the problem of writing a card game, the implementation can be greatly simplified through breaking things down into the constituent objects (suit, rank, card, hand, deck) and functionality (deal, shuffle, discard, etc.) Regardless of the game being implemented, the vast majority of the code needs to be written only once. However, there are three major items that that do change from game to game:
1. The evaluation of a hand
2. The composition of a hand (e.g. in blackjack you initially receive two cards whereas in draw poker you receive five)


3. In some cases, which cards are present in the deck
The Assignment

The current version of the assignment broken up into two major parts. The first part consists of a document having several sections that leads you systematically through the development process. It first requires you to develop the Rank, Suit, Card and Deck classes and implement the CardDeckDemo program that demonstrates that Card objects are successfully being instantiated and can be processed via the Deck. The second section requires you to develop the Hand superclass and implement both thesubclass DumbGameHand and the DumbGame program that exercises the methods of the Card and Hand classes. The third section illustrates the benefit of reusable code by having students implement the Beginner's Blackjack program; this portion of the assignment requires the development of only a new subclass of Hand and a new GUI interface; everything else is based on previously written code, which is rather nifty! The specifications for the Card, Deck, Hand, Rank, and Suit classes are all provided through the use of Javadoc HTML files. Complete source code for the applets is available, as well as stub code for the Card, Deck, Hand, Rank, and Suit classes for you to distribute to your students.
The second part asks you to implement a 'legitimate' card game (my definition of legitimate is that the rules of the game can be found in 'According to Hoyle' or similar reference) that utilizes a standard deck of playing cards - this includes stripped decks (for Euchre) or multiple decks (for Baccarat).
Resources
- Copies of the assignments.
- Javadocs of the Card, Deck, Hand, Rank, and Suit classes.
- Copies of the card images, available either as a zip or as a tar file.
- Sun's documentation on how to write documentation comments for Javadoc.
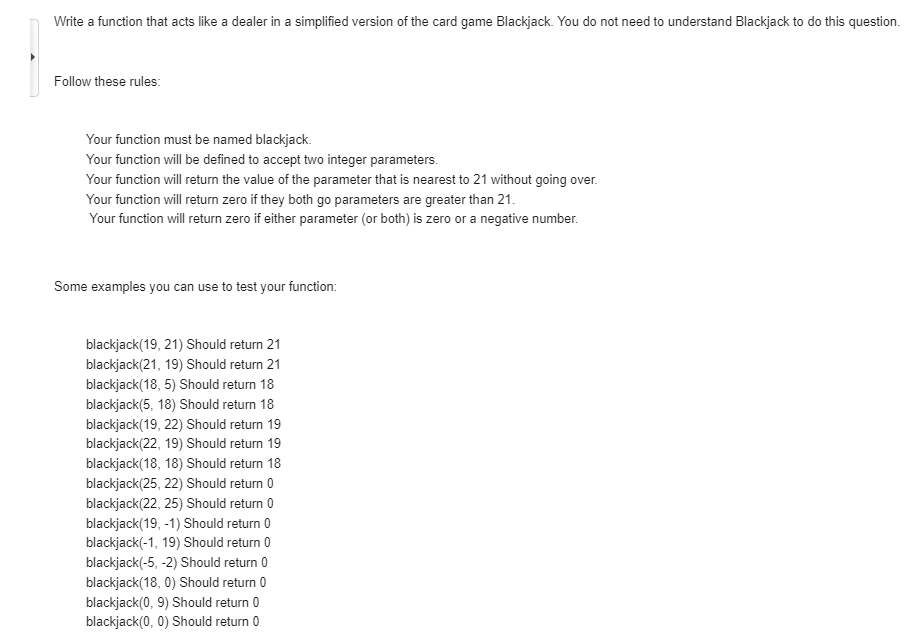
Answers
Blackjack Programming Assignment Definition
the combination of partial code, terrible indenting and german comments probably means you won't get any answers to this...
but, from the description i think the problem is that draw() only shows a result when it finishes. so if you draw 5 things on top of each other in a single draw loop then you'll only see the last one.
Generic remarks:
- I question the usefulness of lines like:
{YouWin = 1 ;
if(YouWin 1 )or
{YouLose = 0;
if (YouLose0){The test after the assignment is totally useless...
In general, avoid the usage of delay(). In the case of
delay(1200);
the sketch is frozen for 1.2 second, which seems long for the user...There is duplicate code in your two branches, you should move it to a method.
I also invite you to read the Technical FAQ. The third article can be relevant. Basically: don't use a loop with delay(), use millis() with some logic.
What i tried to code is a loop which does the exact same thing like the first button but all by itself. Do you have a solution for this? Deadline is 01.04. and I am a bit scared...
you wrote:
What i tried to code is a loop which does the exact same thing like the first button but all by itself.
what is it that the 1st button does?
how is that behaiour triggered?
the advices above are good
moreover your code is not complete and we don't have the images.
can you provide the current version with images as zip via dropbox?